Difference between revisions of "Scripting - OpenKM 5.1"
(→Purge all users trash) |
|||
Line 231: | Line 231: | ||
</source> | </source> | ||
− | == Execute repository stats == | + | === Execute repository stats === |
<source lang="java"> | <source lang="java"> | ||
import com.openkm.module.direct.*; | import com.openkm.module.direct.*; | ||
Line 241: | Line 241: | ||
StatsInfo si = new DirectStatsModule().getDocumentsSizeByContext(systemToken); | StatsInfo si = new DirectStatsModule().getDocumentsSizeByContext(systemToken); | ||
print("StatsInfo: " + si); | print("StatsInfo: " + si); | ||
+ | </source> | ||
+ | |||
+ | === Force datastore garbage collector === | ||
+ | <source lang="java"> | ||
+ | import com.openkm.core.*; | ||
+ | |||
+ | print("Begin"); | ||
+ | new DataStoreGarbageCollector().run(); | ||
+ | print("End"); | ||
</source> | </source> | ||
[[Category: Administration Guide]] | [[Category: Administration Guide]] |
Revision as of 13:45, 5 March 2012
Scripting is an advanced feature - a sort of experimental one - that enables administrators to execute some BeanShell scripts in folders, fired on every notified event ( for example uploading documents ). It could be useful for performing automatic operations at repository level.
Scripting can be set by folder or document. You need to go to Administration > Repository view and browse the repository at low level. Be careful because an error can damage the document repository! When you have located the right place, select Scripting: Set script and a new scripting property will be added to the node. This new property can be edited to set your custom BeanShell code.
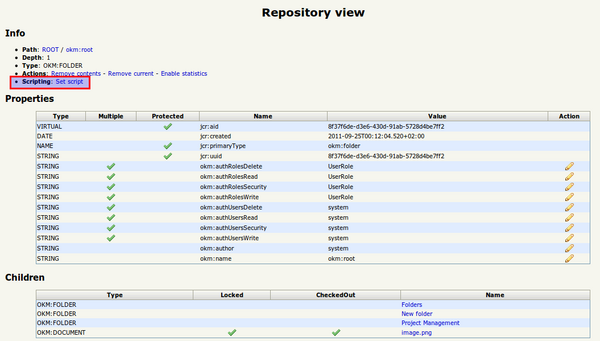
![]() |
OpenKM uses BeanShell. For more information point your browser to http://www.beanshell.org/intro.html. |
BeanShell is a small, free, embeddable Java source interpreter with object scripting language features, written in Java. BeanShell dynamically executes standard Java syntax and extends it with common scripting conveniences such as loose types, commands, and method closures like those in Perl and JavaScript.
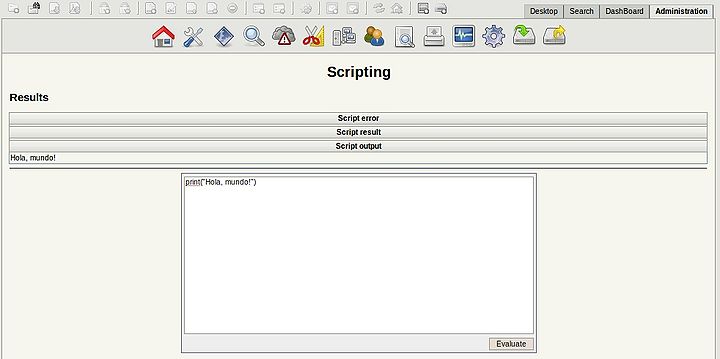
Starting with OpenKM 5.0 you can also execute a custom script when OpenKM starts or stops. To do so, just create a start.bsh (and / or stop.bsh) file in $JBOSS_HOME. For example, we use this feature to create a complete environment (create custom users, register property groups, register workflow) each time the OpenKM demo is reinitialized.
Variable substitution
- java.lang.String eventType - says the event that has fired the script. See below for event types.
- javax.jcr.Session session - users session that executes the script.
- javax.jcr.Node eventNode - node that causes the event.
- javax.jcr.Node scriptNode - node where is stored the script.
Events types
- CREATE_DOCUMENT
- DELETE_DOCUMENT
- SET_DOCUMENT_CONTENT
- SET_DOCUMENT_PROPERTIES
- CHECKOUT_DOCUMENT
- CANCEL_CHECKOUT_DOCUMENT
- CHECKIN_DOCUMENT
- LOCK_DOCUMENT
- UNLOCK_DOCUMENT
- PURGE_DOCUMENT
- CREATE_FOLDER
- DELETE_FOLDER
- PURGE_FOLDER
- CREATE_MAIL
- DELETE_MAIL
- PURGE_MAIL
- ADD_CATEGORY
- REMOVE_CATEGORY
- ADD_KEYWORD
- REMOVE_KEYWORD
Examples
![]() |
If you are using OpenKM 4.1 (or previous), the right package is "es.git.openkm". The package "com.openkm" is valid starting at OpenKM 5.0 |
Automatic renaming of document
String parent = com.openkm.util.FileUtils.getParent(eventNode.getPath());
session.move(eventNode.getPath(), parent+"/renamed_document.doc");
session.save();
Automatic adding of keyword
eventNode.setProperty("okm:keywords", new String[]{"maligno"});
eventNode.save();
Change log level
import org.apache.log4j.*;
Logger log = Logger.getLogger("com.openkm.module");
log.setLevel(Level.DEBUG);
Reload log configuration
This sample work-as-is in OpenKM-LTE.
import org.apache.log4j.*;
import com.openkm.util.*;
String filename = ServerDetector.getHomeDir() + "/lib/log4j.properties";
new PropertyConfigurator().doConfigure(filename, LogManager.getLoggerRepository());
Purge all users trash
Works on OpenKM 5.1 branch.
import com.openkm.api.*;
import com.openkm.core.*;
import com.openkm.bean.*;
String token = JcrSessionManager.getInstance().getSystemToken();
for (Folder trash : OKMFolder.getInstance().getChilds(token, "/okm:trash")) {
print("Trash: " + trash.getPath()+"<br/>");
for (Folder fld : OKMFolder.getInstance().getChilds(token, trash.getPath())) {
print("About to delete folder: " + fld.getPath() + "<br/>");
OKMFolder.getInstance().purge(token, fld.getPath());
}
for (Document doc : OKMDocument.getInstance().getChilds(token, trash.getPath())) {
print("About to delete document: " + doc.getPath() + "<br/>");
OKMDocument.getInstance().purge(token, doc.getPath());
}
for (Mail mail : OKMMail.getInstance().getChilds(token, trash.getPath())) {
print("About to delete mail: " + mail.getPath() + "<br/>");
OKMMail.getInstance().purge(token, mail.getPath());
}
}
Other alternative method:
import javax.jcr.*;
import com.openkm.core.*;
import com.openkm.bean.*;
import com.openkm.module.base.*;
String token = JcrSessionManager.getInstance().getSystemToken();
Session session = JcrSessionManager.getInstance().get(token);
Node trash = session.getRootNode().getNode(Repository.TRASH);
for (NodeIterator it = trash.getNodes(); it.hasNext(); ) {
Node userTrash = it.nextNode();
print("User Trash: " + userTrash.getPath() + "<br/>");
for (NodeIterator itut = userTrash.getNodes(); itut.hasNext(); ) {
Node child = itut.nextNode();
print("About to delete: " + child.getPath() + "<br/>");
if (child.isNodeType(Document.TYPE)) {
BaseDocumentModule.purge(session, child.getParent(), child);
} else if (child.isNodeType(Folder.TYPE)) {
BaseFolderModule.purge(session, child);
}
}
userTrash.save();
}
You can see also a modified version which runs on the older OpenKM 5.0 branch at Forum: Purge trash of all users.
Using Beanshell to move Mails
The Script should check a specific Mail folder ("Docs") and move all the mails in another folder ("okm:root/Mails") in the Taxonomy. This runs as a Cronjob.
import javax.jcr.*;
import com.openkm.core.*;
import com.openkm.bean.*;
import com.openkm.module.*;
String token = JcrSessionManager.getInstance().getSystemToken();
Session session = JcrSessionManager.getInstance().get(token);
Node okmmail = session.getRootNode().getNode(Repository.MAIL);
Node okmroot = session.getRootNode().getNode(Repository.ROOT);
for (NodeIterator it = okmmail.getNodes(); it.hasNext(); ) {
Node userMailFolder = it.nextNode();
if( userMailFolder.getPath().equals("/okm:mail/Docs") ) {
//Check the mail in the "Docs" folder
for (NodeIterator itut = userMailFolder.getNodes(); itut.hasNext(); ) {
Node mail = itut.nextNode();
String name = mail.getName();
//Check the keyword
int available = name.indexOf("al");
if (available != -1){
session.move(mail.getPath(), okmroot.getPath() + "/alFolder/" + mail.getName());
session.save();
}
available = name.indexOf("ka");
if (available != -1){
session.move(mail.getPath(), okmroot.getPath() + "/kaFolder/" + mail.getName());
session.save();
}
}
userMailFolder.save();
}
}
See related forum topic Using Beanshell to move Mails.
After uploading a file, remove all rights - except read - to all users but mantain to uploader
The Script should remove all rights to all users and roles - except read right - and will retain existing privileges of the uploader user.
import java.util.Map;
import com.openkm.api.OKMAuth;
import com.openkm.bean.Permission;
if (eventType.equals("CREATE_DOCUMENT")) {
String userId = session.getUserID();
String path = eventNode.getPath();
OKMAuth oKMAuth = OKMAuth.getInstance();
// All Roles only will continue having read grants
Map hm = oKMAuth.getGrantedRoles(null, path);
for (String roleName : hm.keySet()) {
oKMAuth.revokeRole(null, path, roleName, Permission.WRITE, false);
oKMAuth.revokeRole(null, path, roleName, Permission.DELETE, false);
oKMAuth.revokeRole(null, path, roleName, Permission.SECURITY, false);
}
// All users only will continue having read grants
hm = oKMAuth.getGrantedUsers(null, path);
for (String userName : hm.keySet()) {
if (!session.getUserID().equals(userName)) {
oKMAuth.revokeUser(null, path, userName, Permission.WRITE, false);
oKMAuth.revokeUser(null, path, userName, Permission.DELETE, false);
oKMAuth.revokeUser(null, path, userName, Permission.SECURITY, false);
}
}
}
Execute repository stats
import com.openkm.module.direct.*;
import com.openkm.bean.*;
import com.openkm.core.*;
String systemToken = JcrSessionManager.getInstance().getSystemToken();
print("Token: " + systemToken);
StatsInfo si = new DirectStatsModule().getDocumentsSizeByContext(systemToken);
print("StatsInfo: " + si);
Force datastore garbage collector
import com.openkm.core.*;
print("Begin");
new DataStoreGarbageCollector().run();
print("End");